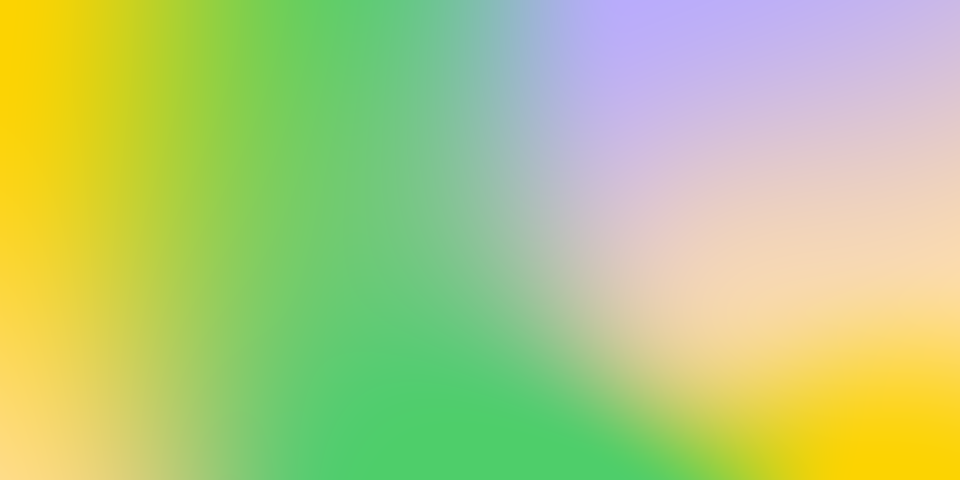
Computational Complexity
Notes from an Udemy Course
Intro
Course: C++ Data Structures & Algorithms + LEETCODE Exercises
Types
Time Complexity
Amount of time used by a program to run completely. But, execution time depends not only on the code itself but also on the capacity of the machine it’s running. So, a program efficiency is not exactly measured in seconds but in terms of complexity’s growth rate as we increase the input size.
Space Complexity
Amount of memory used by a program to run completely. It’s common to see algorithms that uses more memory to reduce execution time or vice-versa.
Symbols
- (uppercase Omega) represents the best case
- (uppercase Theta) represents the average case
- (uppercase Omicron) represents the worst case
notation
Describes the worst-case scenario for an algorithm.
Common complexities
- : Constant Time
- Doesn’t depend on the size of the data set.
- Example: Accessing an array element by its index.
- : Logarithmic Time
- Splits the data in each step (divide and conquer).
- Example: Binary search.
- : Linear Time
- Directly proportional to the data set size.
- Example: Looping through an array.
- : Linearithmic Time
- Splits and sorts or searches data.
- Example: Merge sort, quick sort.
- : Polynomial Time
- Nested loops for each power of n.
- Example: Bubble sort.
- : Factorial Time
- Any algorithm that calculates all permutations of a given array.
- Example: Travelling salesman problem.
Simplification Rules
Drop constants
- ;
- ;
- and so on.
Drop Non-Dominant Terms
- ;
- ;
- and so on.
Show Different Terms for Inputs
If an algorithm has two independents inputs, say and , complexity should be in terms of these variables. For example , , and so on. As and can have different values, .
Cheat Sheet
Here’s a list with some algorithms along with their time and space complexity.
notation
It tells you what to generally expect in terms of me complexity
notation
Describes the best-case scenario for an algorithm.